The roof is closed even though the weather is very nice. I guess the management wants to keep noise level high on a weekday game.
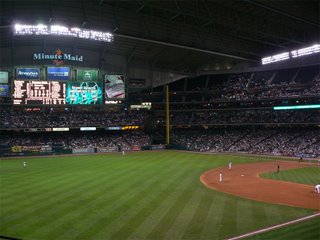
I also see president George Bush senior in the game. See if you spot him in the crowd

so you include the remote scripts with the following statements:
<script type="text/javascript" src="http://remote-domain/test.js"/>
And then when you try to call a method in that test.js script, the browser chokes complaining permission denied.
It doesn't work because we can't fool CDS with this trick!
This time, I try to get the scripts from my local script then attempt to load them in memory using the built-in eval method of javascript 1.4. here is how it works:
<script>
var req;
function loadJS(url)
{
req = false; // branch for native XMLHttpRequest object
if(window.XMLHttpRequest)
{
try { req = new XMLHttpRequest(); } catch(e) { req = false; }
// branch for IE/Windows ActiveX version }
else if(window.ActiveXObject)
{
try
{
req = new ActiveXObject("Msxml2.XMLHTTP");
}
catch(e)
{
try
{
req = new ActiveXObject("Microsoft.XMLHTTP");
}
catch(e) { req = false; }
}
}
if(req)
{
req.onreadystatechange = insertJS;
req.open("GET", url, true); req.send("");
}
}
function insertJS()
{
var code = req.getResponseText;
eval(code);
}
<script/>
So you call this method loadJS('http://remote-domain/test.js') and hope it works. No it doesn't! The same error "permission denied" will popup again.
So I use the previous code to request the scripts from different domain as above. But this time, instead of using the eval method, I create a DOM node to build an inline script tag: